As you may know, Log4j released a patch (actually a couple of patches) recently for a vulnerability [CVE-2021-44228] that was identified in their library. Since a lot of Spring boot applications are out there using Log4j 2.x series. It is better to fix them as soon as possible.
Also, this article is considering you are using Maven for dependency management.
Fixing this in the Spring boot applications is easy. It is just adding a version property for the pom.xml.
As of this date of writing, the latest updated Log4J version is 2.17.1. So the fix is to update the version property with this.
<properties>
<log4j2.version>2.17.1</log4j2.version>
<log4j.version>2.17.1</log4j.version>
</properties>
If you are using BOM, instead of spring boot parent dependency, update it as follows.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-bom</artifactId>
<version>2.17.1</version>
<scope>import</scope>
<type>pom</type>
</dependency>
...
</dependencies>
</dependencyManagement>
Is this enough?
Mostly yes, but maybe Not entirely.
We may be out of the woods since you might have fixed the log4j that is being used within your application code, but what if you have used some other library that is still using the log4j vulnerable version? The best way to find out if you are still using a vulnerable version is to check the dependency tree.
mvn dependency:tree
Since the dependency tree could be quite long and hard to pinpoint the packages, I have used the following trick to identify such dependencies within dependencies.
First, grep the log4j out of the dependency tree
mvn dependency:tree | grep log4j
This will give an output like below.
[INFO] | \- org.apache.logging.log4j:log4j-api:jar:2.17.1:compile
[INFO] | +- log4j:log4j:jar:2.8.2:compile
Notice that, have the proper log4j 2.17 version but still some library is apparently using an older version of log4j. Since it could also be vulnerable we have to exclude this from the parent package.
To find the parent package, we can use the below command.
mvn dependency:tree -Dincludes=log4j
[INFO] com.mycompany.myproject.component1:component1:jar:4.4.0-SNAPSHOT
[INFO] \- com.mycompany.myproject.componentxyz:componentxyz:jar:10.1.15-RELEASE:compile
[INFO] \- log4j:log4j:jar:2.8.2:compile
So, the above output shows the parent packages for the log4j dependency we found earlier.
Notice that, my project is the myproject.component1, and I'm having a dependency within my pom file as below.
<dependency>
<groupId>com.mycompany.myproject</groupId>
<artifactId>componentxyz</artifactId>
<version>10.1.15-RELEASE</version>
</dependency>
Within that, there is a reference for the log4j older version. To fix that, we have to exclude it as below.
<dependency>
<groupId>com.mycompany.myproject</groupId>
<artifactId>componentxyz</artifactId>
<version>10.1.15-RELEASE</version>
<exclusions>
<exclusion>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
</exclusion>
</exclusions>
</dependency>
Check the dependency tree repetitively like this to make sure you're absolutely free of vulnerable dependencies.
P.S.
If your project is getting run time errors after excluding log4j older versions, it could probably be because you excluded a dependency of log4j1.x series. The interfaces are quite different for log4j1.x and log4j2.x. If it is a stable Log4j1.x version without any vulnerabilities, you can just leave it because the above issues are not impacted to the log4j 1.x series.
If you really wanna upgrade that to log4j 2.17.1 then;
If you own the code of this, you will have to update the source code of this dependency to make sure it is using the latest log4j version.
If you don't own it, probably you'll have to reach out to the guys who are developing this for a patch version. There's a good chance they have already updated and released one with a patch.
References:
https://logging.apache.org/log4j/2.x/security.html
https://spring.io/blog/2021/12/10/log4j2-vulnerability-and-spring-boot
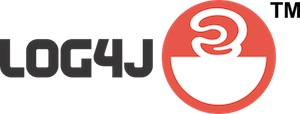 |
Log4j |
Comments
Post a Comment